Broadcasting in Python
- neovijayk
- Jun 13, 2020
- 2 min read
In this article I will explain Broadcasting in Python, related issues, assertion statement. The source for this article is from Course 1 of Deep Learning Specialization on Coursera by Andrew Ng. I find it very useful and want to share some of the important points from the lectures.
Broadcasting in Python
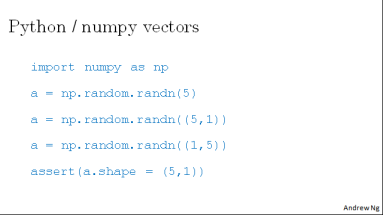
Broadcasting is one of the techniques that you can use to make your Python code run faster.
Always use a reshape command just to make sure that it’s the right column vector or the row vector or whatever you want it to be
Reshape command to make sure that your matrices are the size you need it to be
But wait its having some issues:
There’s also weakness because with broadcasting and this great amount of flexibility, sometimes it’s possible you can introduce very subtle bugs or very strange looking bugs, if you’re not familiar with all of the intricacies of how broadcasting and how features like broadcasting work.
But there’s also weakness because with broadcasting and this great amount of flexibility, sometimes it’s possible you can introduce very subtle bugs or very strange looking bugs, if you’re not familiar with all of the intricacies of how broadcasting and how features like broadcasting work.
So there is an internal logic to these strange effects of Python. It becomes very hard to find bugs if this is the problem.
How to avoid such bugs?
To avoid such bugs Andrew Ng suggested some couple of tips and tricks to eliminate or simplify and eliminate all the strange looking bugs in the code.
Do not use these rank 1 arrays. Example Numpy array with shape (5, )
Instead, if every time you create an array, you commit to making it either a column vector, so this creates a (5,1) vector, or commit to making it a row vector, then the behavior of your vectors may be easier to understand.
So in this case, a.shape is going to be equal to 5,1. And so this behaves a lot like a, but in fact, this is a column vector.
And that’s why you can think of this as (5,1) matrix, where it’s a column vector. And here a.shape is going to be 1,5, and this behaves consistently as a row vector.
assertion statement:
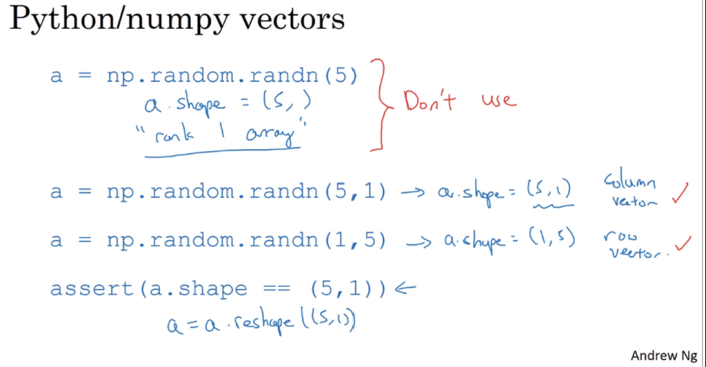
to make sure, in this case, that this is a (5,1) vector
they also help to serve as documentation for your code. So don’t hesitate to throw in assertion statements like this whenever you feel like.
if for some reason you do end up with a rank 1 array, You can reshape this, a equals a.reshape into say a (5,1) array or a (1,5) array so that it behaves more consistently as either column vector or row vector.
Summary of Important points:
don’t use rank 1 arrays. Always use either n by one matrices, basically column vectors, or one by n matrices, or basically row vectors.
Feel free to toss a lot of assertion statements, so double-check the dimensions of your matrices and arrays.
And also, don’t be shy about calling the reshape operation to make sure that your matrices or your vectors are the dimension that you need it to be.
That is it for now. If you like this article please like and subscribe the blog.
Comments